How to Enable Integrated Gallery Search for PageBuilder Editor
There are times when a PageBuilder Editor user would like to add a gallery to a block without having to leave the context of PageBuilder Editor to find and select the video they would like to add A common way to enable this functionality is to create a custom field that allows the user to enter a gallery ID or gallery canonical URL. However, it can be burdensome to search in Photo Center and then copy/paste the ID or URL back into the custom field.
A convenient way to integrate the search with Photo Center’s galleries is to use the searchableField
hook. This hook can be applied to block elements to tell the PageBuilder Editor that users should be able to search Photo Center for a replacement gallery.
Creating a Searchable Gallery
The Photo Center’s galleries search functionality is enabled whenever a Gallery block element uses the searchableField
hook to override custom field values. Let's first define a sample component that uses searchableField
.
The component’s root element must include a style of position: relative for proper button placement in the Editor UI.
Requirements
As a dev dependency, ensure your bundle has
@arc-fusion/cli
that’s version 2.0.9 or higher.
An example feature might look like this:
A Custom Block - /components/features/global/gallery-integration-player-cs.jsx
Note
The following example will produce a block level selection that will appear as custom fields with a dedicated “Select Gallery” button. This is useful if you plan to use multiple content sources for handling various different types of video functionality. This also allows you to select the content source you wish to use.
import PropTypes from 'prop-types' import React from 'react' import { useContent, useEditableContent } from 'fusion:content' const GalleryIntegrationCs = ({ customFields }) => { const { searchableField } = useEditableContent() const { _id } = customFields const { contentService, contentConfigValues } = _id const content = useContent({ source: contentService, key: { _id: contentConfigValues?._id, published: true } }) if (!content) return null const gallery = content?.promo_items?.basic?.additional_properties return ( <div className="feature-listing flex" {...searchableField('_id', 'gallery', { contentSource: 'galleries' })}> <img src={gallery?.originalUrl} width="560" height="315" alt={gallery?.originalName} /> </div> ) } GalleryIntegrationCs.propTypes = { customFields: PropTypes.shape({ _id: PropTypes.contentConfig('feeds').tag({ name: 'Schema' }) }) } GalleryIntegrationCs.label = { en: 'Gallery Search - Content Source' } GalleryIntegrationCs.icon = 'layout-column' export default GalleryIntegrationCs
The values for the searchableField
component hook maps to the following:
Param 1: This maps to the component’s custom field ID associated with the contentConfig.
Param 2: The type of content integration to associate with the contentConfig. In this case the value
gallery
will allow the component to show the gallery search integration.Param 3: An object, which at this time only takes a
contentSource
prop. The value should be the name of the content source for the editor preview to display the story.
This will produce the following selection display in Block Details panel found in Curate Workspace in PageBuilder Editor:
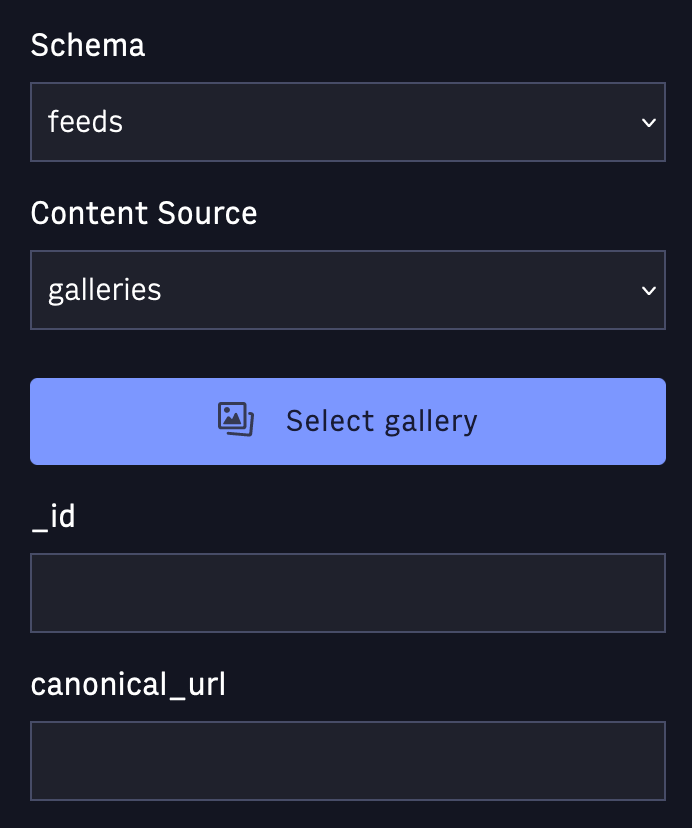
Content Source - /content/sources/story-search.js
const resolve = function resolve(key) { const website = key['arc-site'] || 'islands' const _id = key['_id'] return `/content/v4/galleries/?_id=${_id}&website=${website}` } module.exports = { resolve, schemaName: 'feeds', params: { _id: 'text', canonical_url: 'text' }, searchable: 'gallery' }
This feature applies the searchableField
hook to a top level component, which allows the PageBuilder Editor to replace the desired custom fields with the content provided by the Content API. The input to the searchableField
hook is an Object that contains a mapping of custom fields to the desired values from Photo Center’s galleries. In this example, when a gallery is selected, the gallery _id
custom field will be replaced with the value of the gallery's ID.